tower
import java.io.*;public class Tower{ public static void Tower(int n,char frompg,char topg,char auxpg) { if(n==1) System.out.println("Move disc 1 "+frompg+" --> "+topg); else { Tower(n-1,frompg,auxpg,topg); System.out.println("Move disc "+n+" "+frompg+" --> "+topg); Tower(n-1,auxpg,topg,frompg); } } public static void main(String[] args)throws IOException { int n; BufferedReader keyboard =new BufferedReader(new InputStreamReader(System.in)); System.out.println("請輸入n個tower:"); n=Integer.parseInt(keyboard.readLine()); char X='X',Y='Y',Z='Z'; if(n==0) System.out.println("請重新輸入"); else Tower(n,X,Y,Z); }}
lab-0102
import java.io.*; public class lab_0102 { public static void main(String args[]) throws IOException { double[] num = new double[5]; int a, b; double temp; System.out.println("Enter some number:"); for (a = 0; a <>
BufferedReader input = new BufferedReader(new InputStreamReader(
System.in));
num[a] = Integer.parseInt(input.readLine());
}
for (a = 0; a <>
for (b = 0; b <>
if (num[a] > num[b]) {
temp = num[a];
num[a] = num[b];
num[b] = temp;
}
}
}
for (a = 0; a <>
System.out.print("" + num[a] + " ");
}
System.out.println("");
System.out.println("Largest to Smallest.");
System.exit(0);
}
}
lab_2
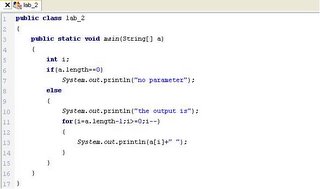
測試結果
Homework 12.19.2005
public class EqualArrays { public static void main(String[] args) { int[] a = {1, 9, 6, 4, 0, 2, 1, 2}; int[] b = {1, 9, 6, 4, 0, 2, 1, 2}; System.out.println("a=1,9,6,4,0,2,1,2"); System.out.println("b=1,9,6,4,0,2,1,2"); if (equalArrays(a, b)) { System.out.println("a and b are equal "); } else { System.out.println("a and b are not equal "); } } public static boolean equalArrays(int a[], int b[]) { if (a.length != b.length) { return false; } else { int i = 0; while (i <>
if (a[i] != b[i]) {
return false;
}
i++;
}
}
return true;
}
}
複數相加
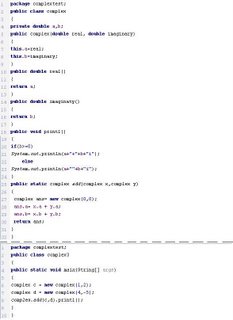
結果
Homework 12-12-2005 Counter II
package javacode2;public class Counter{public Counter(){count = 0;}public void increase(){count++;}public void decrease(){if(count >=1)count--;elsecount = 0;}public int getVasue(){return count;}public void printcount(){System.out.println("counter count is " + count);}public boolean equals(Counter otherCounter){return this.count == otherCounter.count;}public void resetToZero(){count = 0;}public String toString(){return Integer.toString(count) ;}package javacode2;class CounterTest{public static void main(String[] args){Counter counter1 = new Counter();Counter counter2 = new Counter();counter1.increase();counter2.increase();if (counter1.equals(counter2)){System.out.println( counter1 + " is equal to " + counter2);}else{System.out.println( counter1 + " is not equal to " + counter2);}counter2.increase();counter1.decrease();if (counter1.equals(counter2)){System.out.println( counter1 + " is equal to " + counter2);}else{System.out.println( counter1 + " is not equal to " + counter2);}}}
Lab 12-12
public class Fibonacci { public static double n0 = 1, n1 = 1, sum = 0; public static double next() { sum = n0 + n1; n0 = n1; n1 = sum; return sum; } public static void main(String[] args) { for (int i = 0; i <>
Fibonacci.next();
System.out.println("F(" + (i + 2) + ") = " + sum);
}
}
}
Homework 12-5-2005
package bbb; public class temperature { private double degree; private char scale; public temperature() { this.degree=0; this.scale='c'; } public temperature(double degree) { this.degree=degree; this.scale='c'; } public temperature(char scale) { this.degree=0; if(scale=='f'||scale=='F') { this.scale='F'; } else if(scale=='c'||scale=='C') { this.scale='C'; } else { System.out.println("error scale and please scale set C"); this.scale='C'; } } public temperature(double degree,char scale) { this.degree=degree; if(scale=='f'||scale=='F') { this.scale='F'; } else if(scale=='c'||scale=='C') { this.scale='C'; } else { System.out.println("error scale and please scale set C"); this.scale='C'; } } public double get_temperatureC() { double degreesC=0; if(scale=='C') { degreesC=degree; } else if(scale=='F') { degreesC = (degree -32 )*5/9; } return degreesC; } public double get_temperatureF() { double degreesF=0; if(scale=='F') { degreesF=degree; } else if(scale=='C') { degreesF = (degree *9/5 )+32; } return degreesF; } public void setdegree(double degree) { this.degree=degree; } public void setScale(char scale) { if(scale=='f'||scale=='f') { this.scale='F'; } else if(scale=='c'||scale=='C') { this.scale='C'; } else { System.out.println("Error scale and scale no change"); } } public void setScale(double degree,char scale) { if(scale=='f'||scale=='f') { this.scale='F'; } else if(scale=='c'||scale=='C') { this.scale='C'; } else { System.out.println("Error scale and scale no change"); } this.degree=degree; } public boolean isequals(temperature othertemp) { if (this.scale == 'C' && this.degree == othertemp.get_temperatureC()) { return true; } else if (this.scale == 'F' && this.degree == othertemp.get_temperatureF()) { return true; } else { return false; } } public boolean isgreaterthan(temperature otherTemp) { if (this.scale == 'C' && this.degree >= otherTemp.get_temperatureC()) { return true; } else if (this.scale == 'F' && this.degree >= otherTemp.get_temperatureF()) { return true; } else { return false; } } public boolean islessthan(temperature otherTemp) { if (this.scale == 'C' && this.degree <= otherTemp.get_temperatureC()) { return true; } else if (this.scale == 'F' && this.degree <= otherTemp.get_temperatureF()) { return true; } else { return false; } } public String toString() { return Double.toString(degree) + scale; } } ---------------------------------------------------------------------------------------------- package bbb; public class temperature_b { public static void main(String[] args) { temperature cloudC = new temperature(0.0, 'C'); temperature cloudF = new temperature(32.0, 'F'); temperature hotC = new temperature(100.0); hotC.setScale('C'); temperature hotF = new temperature(212.0); hotF.setScale('F'); temperature degreeC = new temperature(); degreeC.setScale(-40.0, 'C'); temperature degreeF = new temperature(); degreeF.setScale('F'); degreeF.setdegree( -40.0); System.out.println("cloudC = " + cloudC ); System.out.println("cloudF = " + cloudF ); System.out.println("hotC = " + hotC ); System.out.println("hotF = " + hotF ); System.out.println("degreeC = " + degreeC + " , " + degreeC.get_temperatureF() + "F"); System.out.println("degreeF = " + degreeF + " , " + degreeF.get_temperatureC() + "C"); System.out.print("Test isequals"); System.out.println(cloudC + " = " + degreeC + " ? " + cloudC.isequals(degreeC)); System.out.println(cloudC + " = " + cloudF + " ? " + cloudC.isequals(cloudF)); System.out.println(cloudF + " = " + degreeC + " ? " + cloudF.isequals(degreeC)); System.out.println(cloudF + " = " + cloudC + " ? " + cloudF.isequals(cloudC)); System.out.print("Test islessthan"); System.out.println(cloudC + " <= " + degreeC + " ? " + cloudC.islessthan(degreeC)); System.out.println(cloudC + " <= " + hotF + " ? " + cloudC.islessthan(hotF)); System.out.println(cloudF + " <= " + degreeC + " ? " + cloudF.islessthan(degreeC)); System.out.println(cloudF + " <= " + hotC + " ? " + cloudF.islessthan(hotC)); System.out.print("Test isgreaterthan"); System.out.println(cloudC + " >= " + hotC + " ? " +cloudC.isgreaterthan(hotC)); System.out.println(cloudC + " >= " + degreeF + " ? " + cloudC.isgreaterthan(degreeF)); System.out.println(cloudF + " >= " + hotF + " ? " + cloudF.isgreaterthan(hotF)); System.out.println(cloudF + " >= " + degreeF + " ? " + cloudF.isgreaterthan(degreeF)); } }
Constructor
package aaaa; import java.io.*; public class Date { private String month; private int day; private int year; public Date ( ) { month = "January"; day = 1; year = 1000; } public Date (int monthInt, int day, int year) { setDate(monthInt, day, year); } public Date (String monthString, int day, int year) { setDate(monthString, day, year); } public Date (int year) { setDate(1, 1, year); } public Date (Date aDate) { if (aDate == null) { System.out.println("Fatal Error."); System.exit(0); } month = aDate.month; day = aDate.day; year = aDate.year; } public void setDate(int monthInt, int day, int year) { if (dateOK(monthInt, day, year)) { this.month = monthString(monthInt); this.day = day; this.year = year; } else { System.out.println("Fatal Error"); System.exit(0); } } public void setDate(String monthString, int day, int year) { if (dateOK(monthString, day, year)) { this.month = monthString; this.day = day; this.year = year; } else { System.out.println("Fatal Error"); System.exit(0); } } public void setDate(int year) { setDate(1, 1, year); } public void setYear(int year) { if ( (year <> 9999) ) { System.out.println("Fatal Error"); System.exit(0); } else this.year = year; } public void setMonth(int monthNumber) { if ((monthNumber <= 0) || (monthNumber > 12)) { System.out.println("Fatal Error"); System.exit(0); } else month = monthString(monthNumber); } public void setDay(int day) { if ((day <= 0) || (day > 31)) { System.out.println("Fatal Error"); System.exit(0); } else this.day = day; } public int getMonth( ) { if (month.equals("January")) return 1; else if (month.equals("February")) return 2; else if (month.equalsIgnoreCase("March")) return 3; else if (month.equalsIgnoreCase("April")) return 4; else if (month.equalsIgnoreCase("May")) return 5; else if (month.equals("June")) return 6; else if (month.equalsIgnoreCase("July")) return 7; else if (month.equalsIgnoreCase("August")) return 8; else if (month.equalsIgnoreCase("September")) return 9; else if (month.equalsIgnoreCase("October")) return 10; else if (month.equals("November")) return 11; else if (month.equals("December")) return 12; else { System.out.println("Fatal Error"); System.exit(0); return 0; } } public int getDay( ) { return day; } public int getYear( ) { return year; } public String toString( ) { return (month + " " + day + ", " + year); } public boolean equals(Date otherDate) { return ( (month.equals(otherDate.month)) && (day == otherDate.day) && (year == otherDate.year) ); } public boolean precedes(Date otherDate) { return ( (year < year ="="">
(year == otherDate.year && month.equals(otherDate.month) && day <>
}
public void readInput( )throws IOException
{
boolean tryAgain = true;
BufferedReader keyboard = new BufferedReader(new InputStreamReader(System.in));
while (tryAgain)
{
System.out.println("Enter month, day, and year.");
System.out.println("Do not use a comma.");
String monthInput = keyboard.readLine( );
int dayInput = Integer.parseInt(keyboard.readLine());
int yearInput = Integer.parseInt(keyboard.readLine());
if (dateOK(monthInput, dayInput, yearInput) )
{
setDate(monthInput, dayInput, yearInput);
tryAgain = false;
}
else
System.out.println("Illegal date. Reenter input.");
}
}
private boolean dateOK(int monthInt, int dayInt, int yearInt)
{
return ( (monthInt >= 1) && (monthInt <= 12) &&
(dayInt >= 1) && (dayInt <= 31) &&
(yearInt >= 1000) && (yearInt <= 9999) );
}
private boolean dateOK(String monthString, int dayInt, int yearInt)
{
return ( monthOK(monthString) &&
(dayInt >= 1) && (dayInt <= 31) &&
(yearInt >= 1000) && (yearInt <= 9999) );
}
private boolean monthOK(String month)
{
return (month.equals("January") || month.equals("February") ||
month.equals("March") || month.equals("April") ||
month.equals("May") || month.equals("June") ||
month.equals("July") || month.equals("August") ||
month.equals("September") || month.equals("October") ||
month.equals("November") || month.equals("December") );
}
private String monthString(int monthNumber)
{
switch (monthNumber)
{
case 1:
return "January";
case 2:
return "February";
case 3:
return "March";
case 4:
return "April";
case 5:
return "May";
case 6:
return "June";
case 7:
return "July";
case 8:
return "August";
case 9:
return "September";
case 10:
return "October";
case 11:
return "November";
case 12:
return "December";
default:
System.out.println("Fatal Error");
System.exit(0);
return "Error";
}
}
}
-------------------------------------------------------------------------------------------------
package aaaa;
public class dis414
{
public static void main(String[]args)
{
Date date1=new Date("December", 16, 1770),
date2=new Date(1, 27, 1756),
date3=new Date (1882),
date4=new Date ();
System.out.println("Whose birthday is " + date1 + "?");
System.out.println("Whose birthday is " + date2 + "?");
System.out.println("Whose birthday is " + date3 + "?");
System.out.println("The default date is " + date4 + ".");
}
}
DateSixthTry
package bbb;import java.io.*;public class DateSixthTry { private String month; private int day; private int year; public void setDate(int monthInt, int day, int year) { if (dateOK(monthInt, day, year)) { this.month = monthString(monthInt); this.day = day; this.year = year; } else { System.out.println("Fatal Error"); System.exit(0); } } public void setDate(int year) { setDate(1, 1, year); } private boolean dateOK(int monthInt, int dayInt, int yearInt) { return ((monthInt >= 1) &&amp; (monthInt <= 12) && (dayInt >= 1) &&amp; (dayInt <= 31) && (yearInt >= 1000) &&amp; (yearInt <= 9999)); } private boolean dateOK(String monthString, int dayInt, int yearInt) { return (monthOK(monthString) && (dayInt >= 1) &&amp; (dayInt <= 31) && (yearInt >= 1000) &&amp; (yearInt <= 9999)); } private boolean monthOK(String month) { return (month.equals("January") || month.equals("February") || month.equals("March") || month.equals("April") || month.equals("May") || month.equals("June") || month.equals("July") || month.equals("August") || month.equals("September") || month.equals("October") || month.equals("November") || month.equals("December")); } public String monthString(int monthNumber) { switch (monthNumber) { case 1: return "January"; case 2: return "February"; case 3: return "March"; case 4: return "April"; case 5: return "May"; case 6: return "June"; case 7: return "July"; case 8: return "August"; case 9: return "September"; case 10: return "October"; case 11: return "November"; case 12: return "December"; default: System.out.println("Fatal Error"); System.exit(0); return "Eorror"; } } public void writeOutput() { System.out.println(month + " " + day + ", " + year); } public void setMonth(int month) { if (dateOK(month, day, year)) { this.month = monthString(month); } else { System.out.println("Fatal Error"); System.exit(0); } } public void setMonth(String month) { if (dateOK(month, day, year)) { this.month = month; } else { System.out.println("Fatal Error"); System.exit(0); } }}